KODE
Start your journey towards developing Today!
Introduction
The Government of Kosovo has been supported from the World Bank to implement the Kosovo Digital Economy (KODE) Project. The KODE Project implemented by the Ministry of Economy will finance the critical fundamentals needed for digital transformation and Policy fulfillment. It will provide high speed broadband infrastructure and support access to labor markets, new sources of knowledge, and public services to households and institutions in Project areas. At the national level, the Project will train and connect youth to employment opportunities through YOU Program and improve access to knowledge sources, including to better reaching and collaborations opportunities to High Educational Institutions.
YOU Program will finance provision of training for young people and their connection to working opportunities. The activities will primarily cater to unemployed or underemployed young men and women to increase their ability to compete in relevant segments of work. The Program will skill beneficiaries to perform IT and IT‐enabled services. It is expected that through this training the beneficiaries will increase their employability in the local ICT market.
iOS Application Development
iOS Development
Introduction
This iOS Development program will prepare you to publish your first iOS app, whether you’re already programming or just beginning. As you master the Swift programming language
and create a portfolio of apps to showcase your skills, you’ll benefit from detailed code reviews, valuable career advice, and coaching from professional iOS developers. You will start by learning the basics of iOS app development using the Swift programming language and Xcode, Apple's development environment. You'll develop your first iOS apps using layouts, views, UIKit, and more. Then, you’ll progress to build more complex and advanced applications, using networking, and will be ready to publish your capstone project to the App Store.
Prerequisites
To develop iOS apps, you need a Mac computer running the latest version of Xcode. Xcode is Apple’s IDE (Integrated Development Environment) for both Mac and iOS apps. Xcode is the graphical interface you'll use to write iOS apps. Xcode includes the iOS SDK, tools, compilers, and frameworks you need specifically to design, develop, write code, and debug an app for iOS. For native mobile app development on iOS, Apple suggests using the modern Swift programming language.
Android Application Development
Android Development
Introduction
Developing applications for Android™ systems requires basic knowledge of Java programming language. This course that focuses on the fundamentals of Java programming language, its framework, syntax, and paradigm. The course will focus on object-oriented programming and techniques which are mainly used in Android software development kit (SDK). It will provide the basic tools and skills to ensure a smooth start with Android application development. This is a crucial course for any non-Java programmer planning to learn the development of Android applications, though it is not mapped to any exam.
Course Prerequisites
This course is designed for software developers or anyone interested in building Android applications. However, computer programming experience in any language is a required prerequisite in order to benefit from this course.
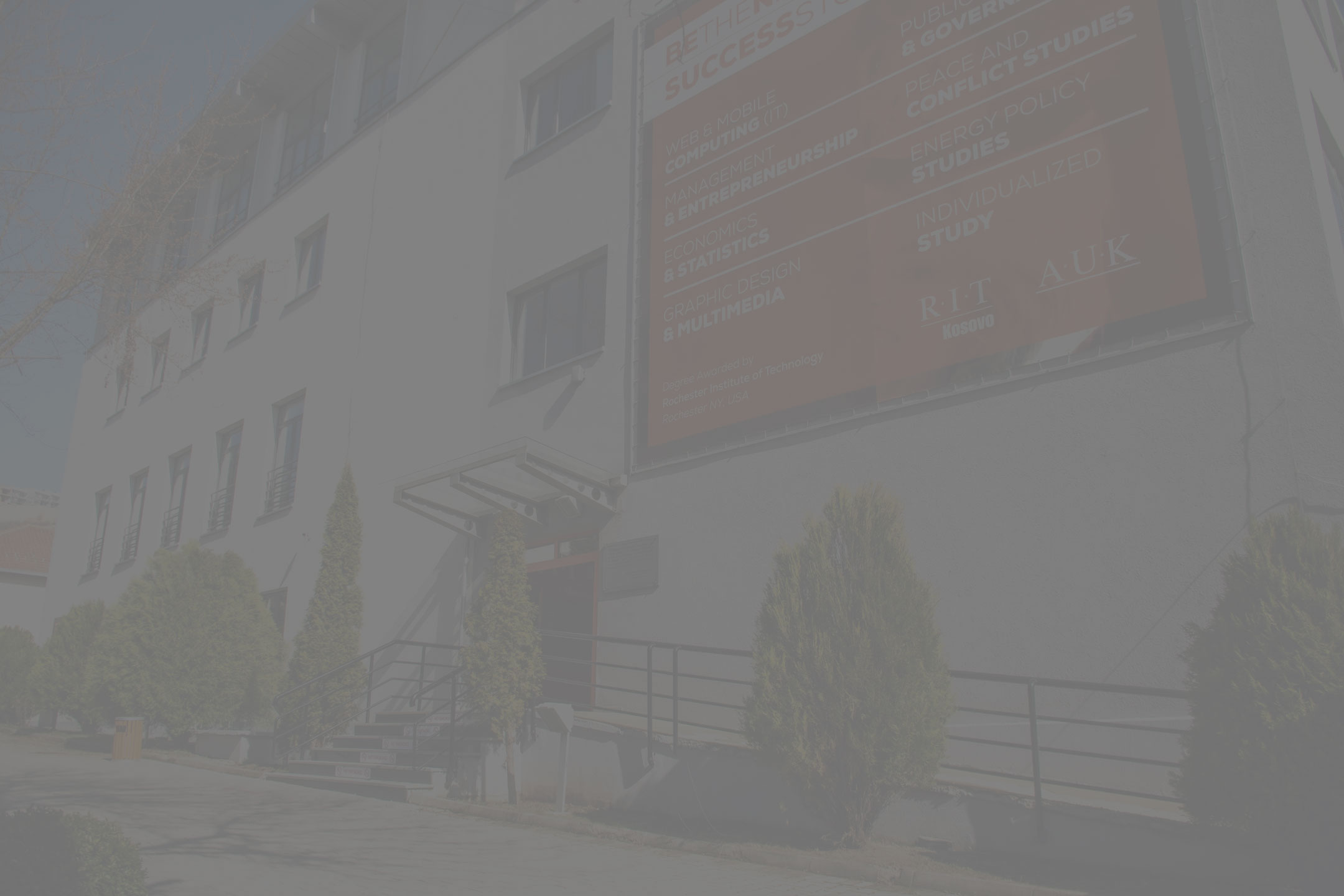
iOS Application Development
iOS Development
Introduction
This iOS Development program will prepare you to publish your first iOS app, whether you’re already programming or just beginning. As you master the Swift programming language
and create a portfolio of apps to showcase your skills, you’ll benefit from detailed code reviews, valuable career advice, and coaching from professional iOS developers. You will start by learning the basics of iOS app development using the Swift programming language and Xcode, Apple's development environment. You'll develop your first iOS apps using layouts, views, UIKit, and more. Then, you’ll progress to build more complex and advanced applications, using networking, and will be ready to publish your capstone project to the App Store.
Prerequisites
To develop iOS apps, you need a Mac computer running the latest version of Xcode. Xcode is Apple’s IDE (Integrated Development Environment) for both Mac and iOS apps. Xcode is the graphical interface you'll use to write iOS apps. Xcode includes the iOS SDK, tools, compilers, and frameworks you need specifically to design, develop, write code, and debug an app for iOS. For native mobile app development on iOS, Apple suggests using the modern Swift programming language.
Android Application Development
Android Development
Introduction
Developing applications for Android™ systems requires basic knowledge of Java programming language. This course that focuses on the fundamentals of Java programming language, its framework, syntax, and paradigm. The course will focus on object-oriented programming and techniques which are mainly used in Android software development kit (SDK). It will provide the basic tools and skills to ensure a smooth start with Android application development. This is a crucial course for any non-Java programmer planning to learn the development of Android applications, though it is not mapped to any exam.
Course Prerequisites
This course is designed for software developers or anyone interested in building Android applications. However, computer programming experience in any language is a required prerequisite in order to benefit from this course.
The Youth Online and Upward (YOU) program is implemented by the KODE Project, in partnership with the Ministry of Economy and supported by the World Bank.
All Trainings are Complimentary / Free of charge for the successful applicants.